CONTENTS
C++ List Alphabetizing: 3 Easy Methods for Sorting
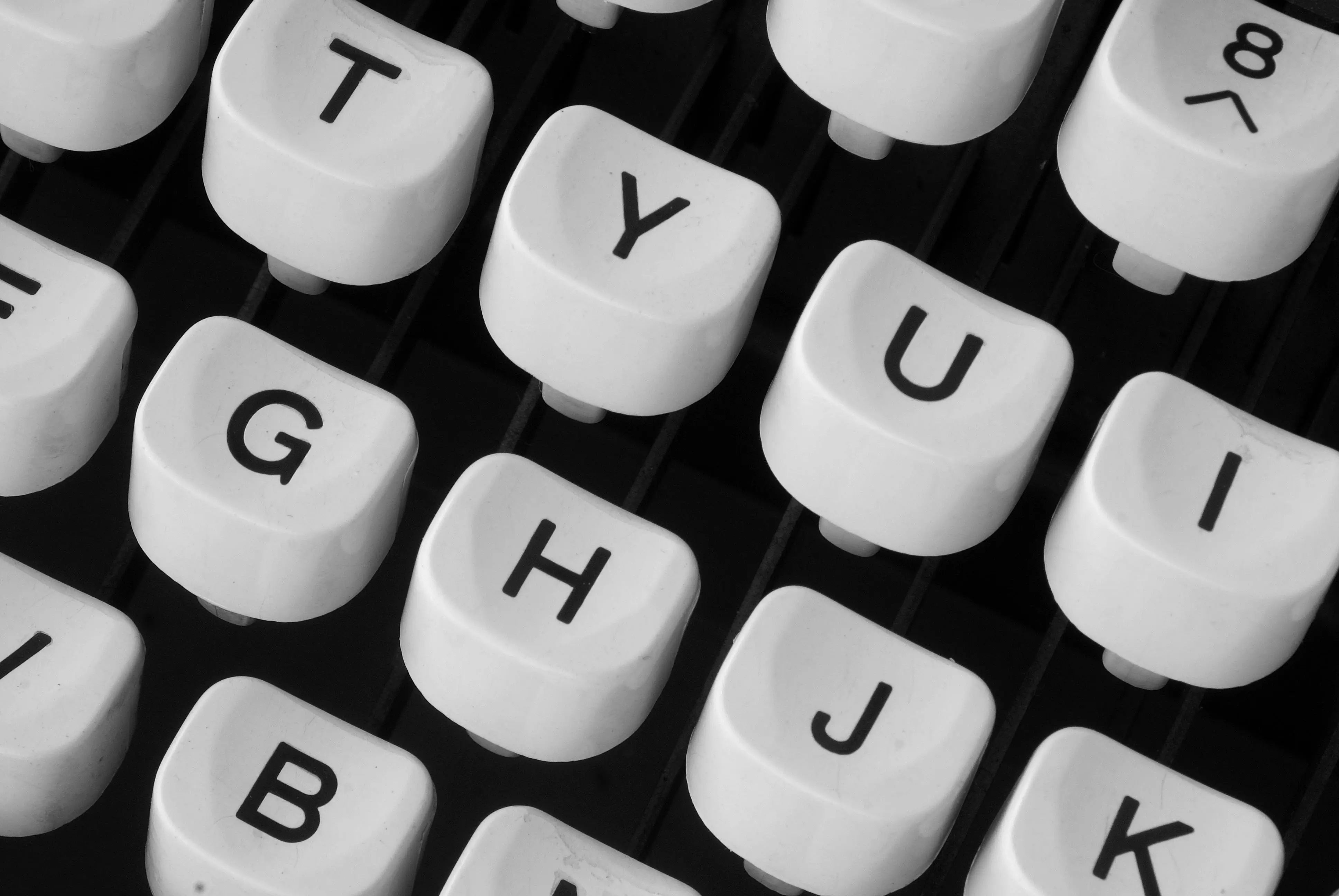
Alphabetizing a list not only enhances data accessibility but also simplifies management. C++ provides several methods to achieve this organizational feat, offering solutions for a diverse range of programming needs. Whether you're working on a personal project, a professional application, or a school assignment, mastering the art of sorting and ordering data is essential.
C++ presents a variety of robust approaches to alphabetizing lists. Whether you seek to sort a list in place, create a new sorted list, or tackle complex alphabetical ordering with special characters, C++ has the tools to address your requirements. In this comprehensive guide, we’ll explore three primary methods for sorting lists alphabetically: using the std::sort()
algorithm, leveraging custom comparators
, and utilizing the std::set
container.
By mastering these techniques, you can ensure that your lists are consistently and accurately alphabetized, facilitating efficient data management and access. Let’s delve into each method and examine how you can implement them in your C++ projects.
Using the std::sort() Algorithm
The std::sort()
algorithm, part of the C++ Standard Library, is a powerful tool for sorting sequences, including arrays and standard containers like vectors. This algorithm rearranges the elements of the container in ascending order by default but can be customized for other orderings. It is an efficient and versatile solution for most sorting needs.
Example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<std::string> names = {"John", "Alice", "Bob", "Charlie"};
// Sorting the vector in alphabetical order
std::sort(names.begin(), names.end());
// Output the sorted vector
for (const auto& name : names) {
std::cout << name << " ";
}
std::cout << std::endl;
return 0;
}
// Output
Alice Bob Charlie John
The std::sort()
algorithm rearranges the elements of the names vector in place, resulting in an alphabetically sorted vector. This method is efficient and does not require additional memory allocation. However, it modifies the original container, which may not be desirable in certain situations.
Using Custom Comparators
For scenarios requiring custom sorting criteria, C++ allows you to define custom comparators. These comparators can be used with sorting algorithms like std::sort()
to impose specific ordering rules on elements.
Example:
#include <iostream>
#include <vector>
#include <algorithm>
bool customCompare(const std::string& a, const std::string& b) {
return a.length() < b.length(); // Sort by length
}
int main() {
std::vector<std::string> names = {"John", "Alice", "Bob", "Charlie"};
// Sorting the vector using a custom comparator
std::sort(names.begin(), names.end(), customCompare);
// Output the sorted vector
for (const auto& name : names) {
std::cout << name << " ";
}
std::cout << std::endl;
return 0;
}
// Output
Bob John Alice Charlie
TIn this example, a custom comparator function customCompare
is defined to sort the names vector based on the length of the strings. The std::sort()
algorithm is invoked with this custom comparator, resulting in a vector sorted by string length.
Utilizing the std::set Container
For scenarios where uniqueness and automatic sorting are desired, the std::set
container in C++ offers an elegant solution. std::set
automatically maintains its elements in sorted order and ensures that each element is unique.
Example:
#include <iostream>
#include <set>
int main() {
std::set<std::string> names = {"John", "Alice", "Bob", "Charlie"};
// Output the sorted set
for (const auto& name : names) {
std::cout << name << " ";
}
std::cout << std::endl;
return 0;
}
// Output
Alice Bob Charlie John
The elements of the names set are automatically sorted alphabetically by default. Additionally, since std::set
does not allow duplicate elements, the set automatically eliminates duplicates, resulting in a unique, sorted collection.
Are C++’s Sorting Algorithms Stable?
In C++, the stability of sorting algorithms can vary depending on the implementation and context. A stable sorting algorithm maintains the relative order of equal elements.
- std::sort() Algorithm: The stability of the
std::sort()
algorithm is implementation-dependent. In many standard library implementations,std::sort()
is stable, ensuring that the relative order of equal elements is preserved. - Custom Comparators: The stability of sorting using custom comparators depends on how the comparison function is implemented. If the comparison function ensures stability, the sorting algorithm will be stable.
Understanding the stability of sorting algorithms is crucial for applications where the order of equal elements is significant.
Check your Alphabetizing Algorithm!
Want to check your C++ alphabetical order sorting algorithm? You can check it against our Free Online Alphabetizing Tool Here!
If you're looking for other languages to Alphabetize your list in, change your preferred programming language in the navigation bar on the top!
As always the source code for this lesson will be on the Dev Donut public github!