CONTENTS
C# List Alphabetizing: 3 sorting Methods
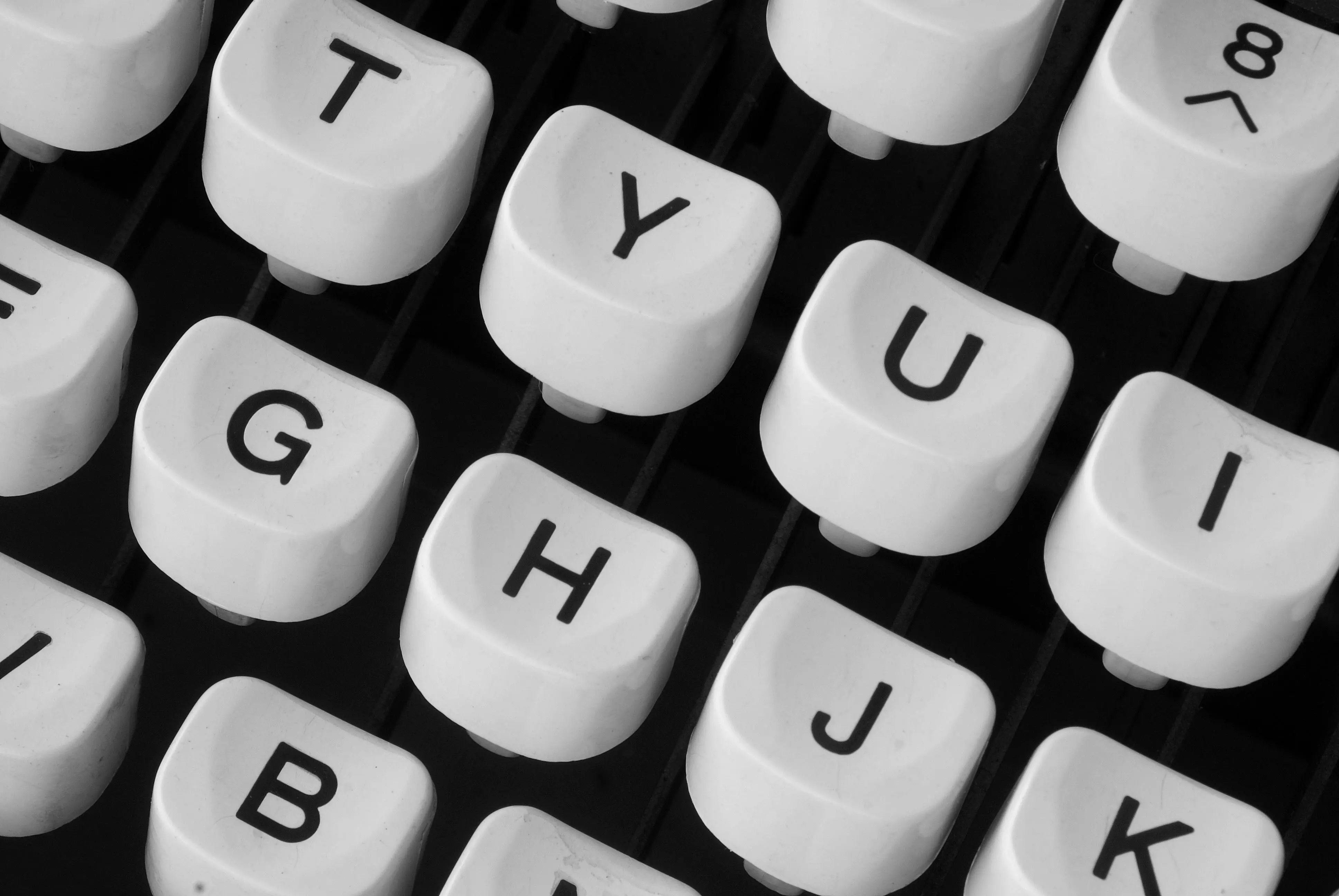
Sorting lists alphabetically in C# is essential for managing names, words, and more. Alphabetizing a list can streamline data management and improve accessibility, and C#—including within the popular Unity game development environment—offers various methods to achieve this. Perfect for streamlining data management in menus, names, and more. Whether you're working on a personal project, a professional application, or an academic assignment, knowing how to efficiently sort and order your data is vital.
C# provides multiple robust ways to alphabetize lists. Whether you need to sort a list in place, create a new sorted list, or handle complex alphabetical ordering with special characters, C# has the tools you need. In this guide, we’ll explore three primary methods for sorting lists alphabetically: using the List<T>.Sort()
method, leveraging LINQ’s OrderBy
method, and implementing custom sorting with the Comparer<T>
class.
By mastering these techniques, you can ensure that your lists are always perfectly alphabetized, making your data easy to manage and access. Let's dive into each method and see how you can implement them in your C# projects.
Using the List.Sort() Method
The List<T>.Sort()
method is a straightforward way to sort a list in C#. This method modifies the original list, arranging its items in alphabetical order. It’s quick and effective for most basic sorting needs.
Example:
using System;
using System.Collections.Generic;
public class SortExample
{
public static void Main()
{
List<string> names = new List<string> { "John", "Alice", "Bob", "Charlie" };
// Sorting the list in alphabetical order
names.Sort();
// Output the sorted list
Console.WriteLine(string.Join(", ", names));
}
}
// Output
Alice, Bob, Charlie, John
The List<T>.Sort()
method sorts the names list in place, updating the original list with the sorted order. This method is efficient as it doesn’t require additional memory to create a new list. However, since List<T>.Sort()
modifies the original list, it may not be suitable if you need to preserve the unsorted version.
Using LINQ’s OrderBy Method
LINQ (Language Integrated Query) offers another useful way to sort a list. The OrderBy
method creates a new sorted sequence from the original list, leaving the original list unchanged. This is beneficial when you need to keep the original list intact.
Example:
using System;
using System.Collections.Generic;
using System.Linq;
public class LinqSortExample
{
public static void Main()
{
List<string> names = new List<string> { "John", "Alice", "Bob", "Charlie" };
// Creating a new sorted list using LINQ
var sortedNames = names.OrderBy(name => name).ToList();
// Output the original and sorted lists
Console.WriteLine("Original list: " + string.Join(", ", names));
Console.WriteLine("Sorted list: " + string.Join(", ", sortedNames));
}
}
// Output
Original list: John, Alice, Bob, Charlie
Sorted list: Alice, Bob, Charlie, John
The OrderBy
method generates a new sorted list called sortedNames, while the original names list remains unchanged. This method is particularly useful when the original order of the list needs to be preserved for other operations or comparisons. Additionally, OrderBy
can accept custom sorting logic through a lambda expression or a Comparer<T>
.
Custom Sorting with the Comparer Class
For more sophisticated alphabetical sorting, especially when dealing with special characters or specific cultural rules, the Comparer<T>
class is essential. It allows you to define custom sorting rules by implementing the IComparer<T>
interface.
Example:
using System;
using System.Collections.Generic;
using System.Globalization;
public class CustomSortExample
{
public static void Main()
{
List<string> names = new List<string> { "John", "Álice", "Bob", "Charlie" };
// Using a custom comparer for locale-aware sorting
names.Sort(new CustomComparer());
// Output the sorted list
Console.WriteLine(string.Join(", ", names));
}
public class CustomComparer : IComparer<string>
{
public int Compare(string x, string y)
{
return string.Compare(x, y, true, new CultureInfo("en-US"));
}
}
}
// Output
Álice, Bob, Charlie, John
By implementing the IComparer<T>
interface in the CustomComparer
class and using the Compare
method, the names list is sorted based on locale-specific alphabetical rules. This is extremely useful for correctly ordering names and words that include special characters. The CustomComparer
ensures that the sorted order respects the specified cultural context.
Are C#’s Sorting Algorithms Stable?
In C#, stability in sorting algorithms is guaranteed by default in the standard library’s sorting methods. A stable sorting algorithm maintains the relative order of equal elements.
- List.Sort() Method: This method uses an introspective sort algorithm, which is stable. It maintains the order of elements that are considered equal.
- LINQ’s OrderBy Method: The
OrderBy
method is stable as well, ensuring that the relative order of equal elements is preserved.
Understanding the stability of these sorting methods is important for applications where the order of equal elements matters. C#’s standard library ensures that sorting is stable, providing reliable and predictable results.
Check your Alphabetizing Algorithm!
Want to check your C# alphabetical order sorting algorithm? You can check it against our Free Online Alphabetizing Tool Here!
If you're looking for other languages to Alphabetize your list in, change your preferred programming language in the navigation bar on the top!
As always the source code for this lesson will be on the Dev Donut public github!