CONTENTS
3 Efficient Ways to Sort Arrays Alphabetically in Java
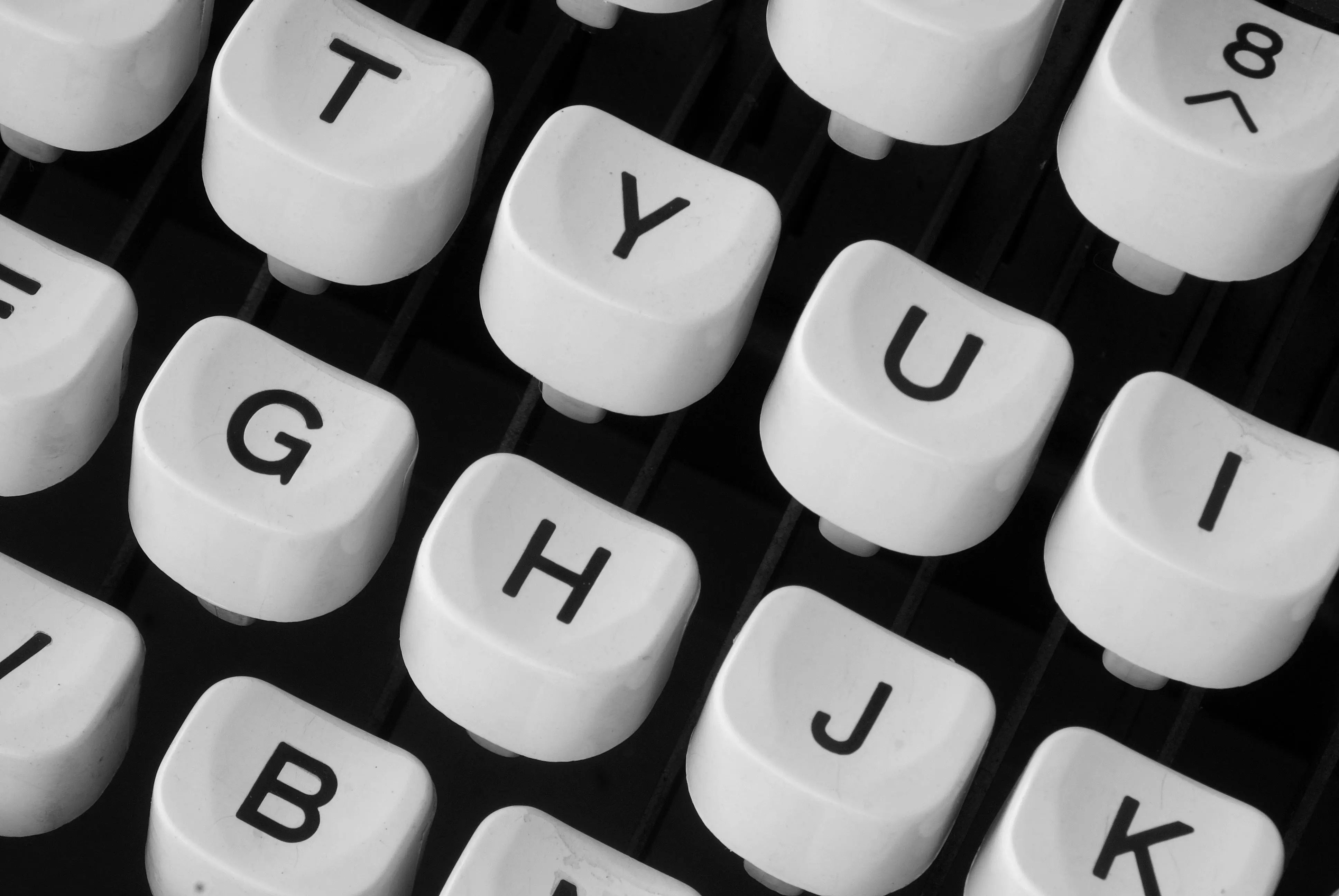
When it comes to managing and organizing data, sorting is an essential task, especially with lists containing names, words, or other text elements. Alphabetizing a list can make it easier to locate and handle items, and Java offers several effective tools to accomplish this. Whether you're tackling a school project, a work assignment, or a personal endeavor, being adept at sorting and ordering your data is crucial.
Java provides several robust methods for alphabetizing lists, thanks to its extensive built-in libraries and functions. Whether you need to sort a list in place, generate a new sorted list, or handle complex alphabetical ordering with special characters, Java has the right tools for the job. This guide will cover three primary techniques for sorting lists alphabetically: using the Collections.sort()
method, the Stream.sorted()
method, and custom sorting with the Collator
class.
By learning these methods, you can keep your lists perfectly alphabetized, making your data more manageable and accessible. Let’s dive into each approach and see how to implement them in your Java projects.
Using the Collections.sort() Method
The Collections.sort()
method offers a simple way to sort a list directly. This method modifies the original list, arranging its items in alphabetical order. It's quick and efficient, ideal when the original order isn't needed.
Example:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class SortExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("John");
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Sorting the list in alphabetical order
Collections.sort(names);
// Output the sorted list
System.out.println(names);
}
}
// Output
[Alice, Bob, Charlie, John]
The Collections.sort()
method sorts the names list in place, updating the original list with the sorted order. This approach is efficient since it doesn't require additional memory to create a new list. However, since Collections.sort()
modifies the original list, it may not be the best choice if you need to preserve the unsorted version. The Collections.sort()
method can also accept a Comparator
if custom sorting is needed.
Using the Stream.sorted() Method
The Stream.sorted()
method is another useful way to sort a list. Unlike Collections.sort()
, Stream.sorted()
creates a new stream with the sorted items, leaving the original list unchanged. This is beneficial when you need to retain the original list.
Example:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class StreamSortExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("John");
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Creating a new sorted list using streams
List<String> sortedNames = names.stream()
.sorted()
.collect(Collectors.toList());
// Output the original and sorted lists
System.out.println("Original list: " + names);
System.out.println("Sorted list: " + sortedNames);
}
}
// Output
Original list: [John, Alice, Bob, Charlie]
Sorted list: [Alice, Bob, Charlie, John]
The Stream.sorted()
method generates a new list called sortedNames, while the original names list remains unchanged. This method is particularly useful when the original order of the list needs to be preserved for other operations or comparisons. Similar to Collections.sort()
, Stream.sorted()
can accept a Comparator for custom sorting.
Custom Sorting with the Collator Class
For more sophisticated alphabetical sorting, especially when dealing with special characters or different languages, the Collator
class is essential. It helps sort strings according to the rules of a specific locale, ensuring the correct order.
Example:
import java.text.Collator;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Locale;
public class CollatorSortExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("John");
names.add("Álice");
names.add("Bob");
names.add("Charlie");
// Setting the locale to a specific setting (e.g., US)
Collator collator = Collator.getInstance(Locale.US);
// Sorting the list using locale-aware sorting
Collections.sort(names, collator);
// Output the sorted list
System.out.println(names);
}
}
// Output
[Álice, Bob, Charlie, John]
By creating a Collator
instance with Collator.getInstance()
and specifying the desired locale, the names list is sorted based on locale-specific alphabetical rules. This is extremely useful for correctly ordering names and words that include special characters. Without setting the appropriate locale, Java’s default sorting behavior may not handle special characters correctly, leading to unexpected orderings. The Collator
class ensures the sorted order is accurate for the specified cultural context.
Are Java’s Sorting Algorithms Stable?
Stability in sorting algorithms is crucial, especially when you need to maintain the relative order of equal elements. In Java, the primary sorting algorithms used in its standard library are stable.
- Collections.sort() Method: This method uses the
TimSort
algorithm, which is a hybrid sorting algorithm derived from merge sort and insertion sort. TimSort is stable, meaning it preserves the order of equal elements as they appeared in the original list. - Stream.sorted() Method: Similar to
Collections.sort()
, theStream.sorted()
method also relies onTimSort
for its sorting mechanism. Therefore, it is stable and maintains the relative order of elements that compare as equal.
Understanding the stability of these sorting methods is important for applications where the order of equal elements matters. Java’s standard library ensures that object sorting remains stable, providing predictable and reliable results.
Check your Alphabetizing Algorithm!
Want to check your Java alphabetical order sorting algorithm? You can check it against our Free Online Alphabetizing Tool Here!
If you're looking for other languages to Alphabetize your list in, change your preferred programming language in the navigation bar on the top!
As always the source code for this lesson will be on the Dev Donut public github!