CONTENTS
3 Easy Ways to Sort Arrays Alphabetically in JavaScript (For Beginners)
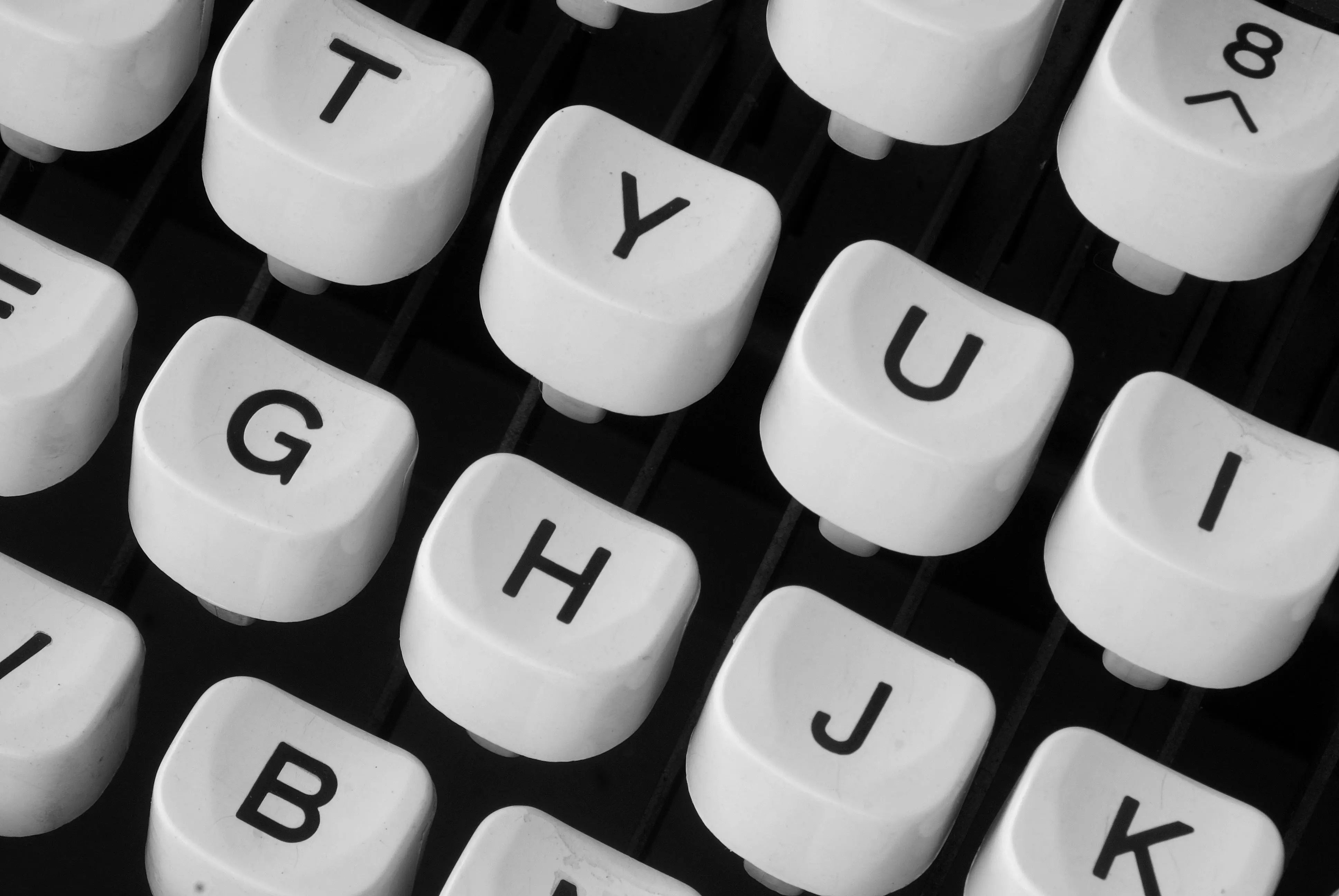
Sorting and organizing data is a fundamental task in many programming endeavors, especially when dealing with lists of names, words, or other text elements. Alphabetizing a list can make it easier to find and manage items, and JavaScript offers a variety of methods to help you do just that. Whether you're working on a personal project, a school assignment, or a professional application, knowing how to efficiently sort and order your data is essential.
JavaScript provides several powerful ways to alphabetize lists. Whether you need to sort a list in place, create a new sorted list, or handle more complex alphabetical ordering with special characters, JavaScript has you covered. In this guide, we'll explore three primary methods for sorting lists alphabetically: using the sort()
method, leveraging locale-aware sorting with localeCompare()
, and creating custom sort functions.
By mastering these techniques, you can ensure that your lists are always in perfect alphabetical order, making your data easy to manage and access. Let's dive into each method and see how you can implement them in your JavaScript projects.
Using the sort() Method
The sort()
method is a straightforward way to sort an array in JavaScript. This method changes the original array directly, arranging its items in alphabetical order. It’s quick and easy, perfect for most basic sorting needs.
Example:
// Create an array of names to sort
const names = ['John', 'Alice', 'Bob', 'Charlie']
// Sorting the array in alphabetical order
names.sort()
// Output the sorted array
console.log(names)
// Output
['Alice', 'Bob', 'Charlie', 'John']
The sort()
method sorts the names array in place, meaning the original array is updated with the sorted order. This method is efficient because it doesn’t require additional memory to create a new array. However, it’s important to note that since sort()
modifies the original array, if you need to keep the unsorted version for any reason, this method might not be the best choice.
Using localeCompare() for Locale-Aware Sorting
For more sophisticated alphabetical sorting, especially if you’re dealing with special characters or different languages, the localeCompare()
method is your friend. It helps you sort strings according to the rules of a specific locale, making sure the order is just right.
Example:
const names = ['John', 'Álice', 'Bob', 'Charlie']
// Sorting the array using locale-aware sorting
names.sort((a, b) => a.localeCompare(b))
// Output the sorted array
console.log(names)
// Output
['Álice', 'Bob', 'Charlie', 'John']
By using the localeCompare()
method inside the sort()
method, the names array is sorted based on locale-specific alphabetical rules. This is super handy for correctly ordering names and words that include special characters. Without specifying the locale, JavaScript’s default sorting behavior might not correctly handle special characters, leading to unexpected orderings. Using localeCompare()
ensures that the sorted order respects the rules of the specified locale.
Custom Sorting with a Comparator Function
For cases where you need even more control over the sorting logic, you can use a custom comparator function. This allows you to define exactly how the elements should be compared and ordered.
Example:
const names = ['John', 'alice', 'Bob', 'Charlie']
// Custom sorting function to handle case-insensitive sorting
names.sort((a, b) => {
const nameA = a.toLowerCase()
const nameB = b.toLowerCase()
if (nameA < nameB)
return -1
if (nameA > nameB)
return 1
return 0
})
// Output the sorted array
console.log(names)
// Output
['alice', 'Bob', 'Charlie', 'John']
The custom comparator function converts both strings to lowercase before comparing them. This ensures that the sorting is case-insensitive, which is useful when you want to sort a list of names without regard to their case. The sort()
method uses the return value of the comparator function to determine the order of the elements. If the function returns a negative value, the first element is considered smaller; if it returns a positive value, the second element is considered smaller; and if it returns zero, the elements are considered equal.
Are JavaScript's Sorting Algorithms Stable?
In JavaScript, the stability of sorting algorithms can depend on the environment and implementation. A stable sorting algorithm maintains the relative order of equal elements. In most modern JavaScript engines, the sort()
method is stable, but it's good to be aware that this can vary:
- V8 Engine (Chrome, Node.js): As of recent versions, the V8 engine (used in Chrome and Node.js) uses a stable sort algorithm.
- SpiderMonkey (Firefox): SpiderMonkey also uses a stable sorting algorithm.
- JavaScriptCore (Safari): JavaScriptCore, used in Safari, has a stable sort implementation.
Generally, you can rely on the stability of sorting in JavaScript for standard use cases. However, for critical applications where stability is essential, always refer to the specific engine’s documentation and testing.
Check your Alphabetizing Algorithm!
Want to check your JavaScript alphabetical order sorting algorithm? You can check it against our Free Online Alphabetizing Tool Here!
If you're looking for other languages to Alphabetize your list in, change your preferred programming language in the navigation bar on the top!
As always the source code for this lesson will be on the Dev Donut public github!