CONTENTS
Alphabetize a List in Python: 3 Easy Methods
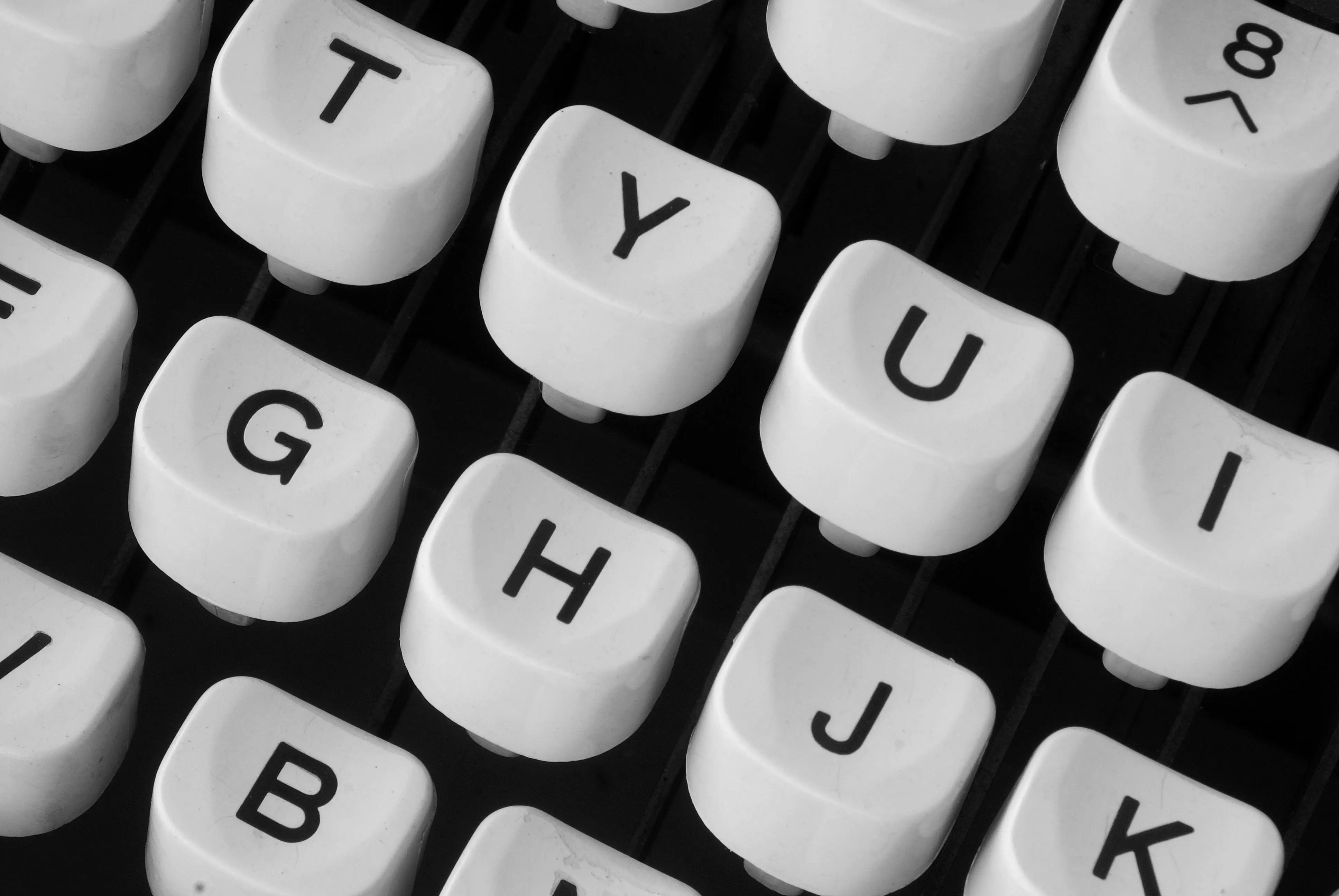
Sorting and organizing data is a common task in many programming projects, especially when you're dealing with lists of names, words, or other textual data. Alphabetizing a list can make it easier to find and manage items, and Python offers some great tools to help you do this. Whether you're working on a school project, a work assignment, or a personal project, knowing how to efficiently sort and order your data is key.
Python, with its powerful built-in functions and modules, provides several straightforward ways to alphabetize a list. Whether you’re looking to sort a list in place, create a new sorted list, or handle more complex alphabetical ordering with special characters, Python 3 has got you covered. In this guide, we'll dive into three primary methods for sorting lists alphabetically: using the sort()
method, the sorted()
function, and custom sorting with the locale
module for more nuanced alphabetical ordering.
By mastering these techniques, you can ensure that your lists are always in perfect alphabetical order, making your data easy to manage and access. Let’s explore each method and see how you can implement them in your Python 3 projects.
Using the sort() Method
The sort()
method is a powerful and efficient way to sort a list in place. This method modifies the original list directly, arranging its elements in ascending order by default. It's particularly useful when you want to sort a list without creating a new one.
Example:
# List of names
names = ['John', 'Alice', 'Bob', 'Charlie']
# Sorting the list in alphabetical order
names.sort()
# Print the sorted list of names
print(names)
# Output
['Alice', 'Bob', 'Charlie', 'John']
The sort()
method sorts the names list in place, meaning the original list is updated with the sorted order. This method is particularly efficient because it doesn’t require additional memory to create a new list. However, it’s important to note that since sort()
modifies the original list, if you need to keep the unsorted version for any reason, this method might not be the best choice. The sort()
method can also accept parameters like reverse=True if you need to sort the list in descending order.
Using the sorted() Function
The sorted()
function is another handy way to sort a list. Unlike sort()
, sorted()
creates a new list that contains the sorted items, leaving the original list unchanged. This is useful when you need to keep the original list intact.
Example:
# List of names
names = ['John', 'Alice', 'Bob', 'Charlie']
# Creating a new sorted list
sorted_names = sorted(names)
# Output the original and sorted lists
print("Original list:", names)
print("Sorted list:", sorted_names)
# Output
Original list: ['John', 'Alice', 'Bob', 'Charlie']
Sorted list: ['Alice', 'Bob', 'Charlie', 'John']
The sorted()
function stores to a new list called sorted_names, while the original names list stays the same. This function is particularly useful when you need to retain the original order of the list for other operations or for comparison purposes. Similar to sort()
, sorted()
can accept parameters like reverse=True to sort the list in descending order, and a key parameter to specify a function to be called on each list element prior to making comparisons.
Custom Sorting with the locale Module
For more advanced alphabetical sorting, especially if you’re dealing with special characters or different languages, the locale
module is your best friend. It helps you sort strings according to the rules of a specific locale, making sure the order is just right.
Example:
import locale
# List of names with special characters
names = ['John', 'Álice', 'Bob', 'Charlie']
# Setting the locale to a specific setting (e.g., 'en_US.UTF-8')
locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
# Sorting the list using locale-aware sorting
sorted_names = sorted(names, key=locale.strxfrm)
# Output the sorted list
print(sorted_names)
# Output
['Álice', 'Bob', 'Charlie', 'John']
By setting the locale with locale.setlocale()
and using locale.strxfrm
as the sorting key, the names list is sorted based on locale-specific alphabetical rules. This is super handy for correctly ordering names and words that include special characters. Without setting the appropriate locale, Python 3’s default sorting behavior may not correctly handle special characters, leading to unexpected orderings.
The locale.strxfrm
function transforms each string to a representation that respects the locale’s sorting rules, ensuring that the sorted order is accurate for the specified cultural context. For more information on locale.strxfrm
check out the official Python3 docs!
Are Python’s Sorting Algorithms Stable?
Yes, Python’s sorting algorithms are stable. This means that when sorting a list, if two items have the same key value, their original order will be preserved in the sorted list. This stability is particularly important when sorting complex data structures, such as lists of tuples or objects, where secondary order might need to be maintained.
Example:
# List of tuples with names and ages
people = [('John', 25), ('Alice', 30), ('Bob', 25), ('Charlie', 35)]
# Sorting by age
people.sort(key=lambda x: x[1])
# Output the sorted list
print(people)
# Output the sorted list
print(sorted_names)
# Output
[('John', 25), ('Bob', 25), ('Alice', 30), ('Charlie', 35)]
In the example above, when sorting by age, 'John' and 'Bob' both have the age of 25. Because Python’s sort is stable, 'John' appears before 'Bob' in the sorted list, preserving their original order relative to each other.
Check your Alphabetizing Algorithm!
Want to check your Python alphabetical order sorting algorithm? You can check it against our Free Online Alphabetizing Tool Here!
If you're looking for other languages to Alphabetize your list in, change your preferred programming language in the navigation bar on the top!
As always the source code for this lesson will be on the Dev Donut public github!